Difference between while and do while loops (code)
Today we’ll do a more technical topic than usual. I’ll write about computing, and particularly about algorithms.
The difference between “while” and “do while” loops seems to interest many people, but the answer wasn’t easy to find on Google. So, here is my quick answer:
As a whole, a loop is a structural part in a source code, allowing developers to repeat an action several times. The main difference between “while” and “do while” is that the latter is executed at least once, even if the condition is false at the beginning.
Don’t worry if this answer isn’t clear enough for you, depending on your coding skills it might be normal. I will explain everything in details now, you’ll get it!
The “while” loop
Definition
In most computer programming languages, a while loop is a control flow statement that allows code to be executed repeatedly based on a given Boolean condition.
Wikipedia
The goal of a loop is to repeat some code lines several times
To limit the number of times the code will be executed, there are several solutions, the “while” loop is one of them
When using “while” loops, we execute the code while the condition is true
If you want, it’s like an “if” structure, with the particularity to come back to the beginning of the “if” when the condition is still true
Syntax
Here, I’ll show you a schematic syntax, just to understand how it works, it’s not a real code
The basic syntax that you probably already learned, looks like this:
WHILE condition DO
//the code here will be executed several times
ENDWHILE
So we have to define a condition, like in conditional statements (if)
Then fill the code to execute several times, if the condition is still true, between WHILE and ENDWHILE
A basic example could be:
Firstname = ""
WHILE Firstname = "" DO
WRITE "What's your firstname?"
READ Firstname
ENDWHILE
This loop allows us to ask the user first name as long as he or she does not give us a valid answer
So the condition is firstname = ""
and it’s true until the user doesn’t type an answer (by only typing Enter for example)
We repeat the lines starting with “READ” and “WRITE” as the long as the first name is not correct
When can you use it?
You probably don’t know this, but I’m a web developer, so I can tell you exactly in which case you can use the “while” loop, rather than another one
At school, we are told that we’ll use it to validate user values, but it’s been a long time since we’ve been using graphical interfaces instead of black and white terminals 🙂
In 99% of the cases, I’m using this expression to do a database request
Generally I don’t know how many values I’ll get in return, so I can’t use a loop to read all the entries
For example, if I need to display all the orders for a specific client, or to process many lines of data returned by a request, etc.
Example
Here is a real example, translated in an algorithmic language, to make it easier to understand (it’s still not a real code sample)
In this example, I will continue with my idea of a database request with the goal to display all the orders of a client
Once translated into a fake language, here is what it could look like:
Result = DatabaseQuery("...") WHILE Result DO NEW_LINE DISPLAY_COLUMN Result.DatePurchased DISPLAY_COLUMN Result.Price DISPLAY_COLUMN Result.OrderStatus CLOSE_LINE ENDWHILE
I hope you now better understand how it works, and what you can really do with a WHILE loop
The “Do While” loop
Definition
In most computer programming languages, a do while loop is a control flow statement that executes a block of code at least once, and then repeatedly executes the block, or not, depending on a given boolean condition at the end of the block.
Wikipedia
It says everything you need to know for an English definition of this loop
So we can move directly to the code part 🙂
Syntax
The basic syntax could look like this (only fake code, like in the previous part):
DO //CODE TO REPEAT WHILE condition
And here is a more explicit example:
Password = “secret”
DO
WRITE “What’s the password?”
READ Input
WHILE Input == Password
We ask the password again and again, until it corresponds to the good one
The rest of the code will not be executed if the user doesn’t know the password
In this example, the boolean condition is “Input == Password”
It’s true only if the entered text corresponds to “secret”
And lines READ and WRITE are always repeated, while this condition is true
You can note here, that these two expressions are executed at least one time
Usage
Hum, here it’s more complex to give you a real example because I never use this loop
I even had difficulties to find the syntax in to do this in my favorites languages
Why?
Because it’s absolutely the same loop as the previous one, everything you do with a “do while” loop, you can write it differently by using a “while” loop
You can scroll up to my example with the first name for the while loop, it’s very similar to the one I gave you here with the password
If I invert the password condition (“Input != Password”) and put it at the beginning of a while loop, it works perfectly
So, I’ll give you an example now, but remember that you will probably never use this loop again after your exam 🙂
Example
The best way I see to use this loop type, is when you need to ask something to the user because you necessarily need to ask something once before he or she can answer
Here is a short example, where the user fills a form:
DO WRITE "*" READ_FIELD "Email" WHILE Email CONTAINS @
This is not obvious, but the goal here is to put a red asterisk near the email field, to indicate a bad value
I look like to many source codes to find a good example, but didn’t find a good one for you, I’m sorry
I hope you at least understand the concept
Differences between loops “while” and “do while”
We’ll now look at the most important differences to remember that you have seen in the previous parts
Number of executions
If you were attentive, you probably note that there is a major difference in the number of times they can be executed
The “While” loop is not necessarily executed at all if the condition is false at the beginning. It can be executed between 0 and an infinity of times
At the opposite, the “Do while” loop is executed at least one time, as we test the condition at the end
You have to think to this difference when you write a code using one of these loops (and it’s probably why teachers continue to explain both, to make you think ^^)
Structures
The code structure is also different
In one case we test the condition immediately at the beginning, and in the other case we execute the lines once before testing the condition
You can also note, that a “while” loop can’t be at the beginning of a code, you have to initialize variables before, in order to have a useful test
However, for the “do while” loop, you can define variables in the loop, that will be used in the condition
Conditions
You may already have noticed this, but you can’t copy and paste a condition from a “while” loop to a “do while” loop, without changing anything
Most of the time you have to revert it
For example:
- WHILE Value <= 10
- DO … WHILE VALUE > 10
Conclusion
We are already at the end of this comparison between these two loops that cause issues to many students: “While” and “Do While” 🙂
Even if I may master my job in a specific language, my algorithmic lessons go back far in time
I hope this article was still useful for you
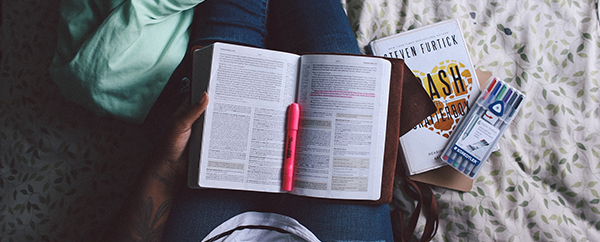
If you want to get more information about algorithms, I highly recommend to lean with an expert, by buying a method that will help you from the basics to complex notions
Algorithmic is a particular subject, where anybody can use a different code, learning on Internet with many sources, in maybe not a good idea
You can find good books on Amazon or find a course on Skillshare or Udemy to find the best place to learn everything from scratch
Find the good option for you, and the good teacher
Good luck!